Diving into OAuth is like opening the door to some seriously important security talk, especially since it's everywhere in today's apps. Whether you're a developer, a security enthusiast, or just curious about how your favorite apps play nice with each other, understanding OAuth is clutch. To keep things organized and ensure you get the most out of this series, here’s a quick agenda of what we’ll be covering:
- Introduction to OAuth
- What the Heck is OAuth?
- OAuth vs. Authentication: What's the Deal?
- Why Was OAuth Born?
- OAuth Actors
- Authorization Grant Types
- Tokens: The VIP Passes
- OAuth Vulnerabilities
- Vulnerabilities Related to Clients, Authorization Servers, Resource Servers, and Tokens
- Using OAuth in Authentication
- OAuth vs. OpenID Connect: Clearing the Confusion
- Risks of Using OAuth as an Authentication Protocol
- Importance of Using the ID Token in OpenID Connect
- Testing Social Logins in Applications
- Introduction to Social Logins
- 10+ Scenarios for Account Takeover (ATO) Related to Social Logins
- Tools and Methods for Testing Social Logins
These real-world examples include everything from poor token validation to account takeover via social login, where chained bugs like deep link abuse or open redirects can lead to full session hijack
Grab your tea, and let's kick things off with Part 1
--( Introduction to OAuth )--
What the Heck is OAuth?
Alright, so picture OAuth as that cool bouncer at a club. You wanna get in (access some resources), but instead of handing over your keys (password), you get a special wristband (token) that says you’re good to go. OAuth is an open-standard protocol that lets apps securely access your info without you spilling your passwords everywhere. It’s all about giving limited access so your data stays safe.
OAuth vs. Authentication: What's the Deal?
Here’s the tea: OAuth and authentication are like peanut butter and jelly—they go together but aren’t the same thing.
- Authentication is about proving who you are. Think logging into your email with your username and password.
- OAuth, on the other hand, is about authorization. It lets one app access your data from another app without sharing your creds. Like letting Instagram post to your Facebook without giving Instagram your Facebook password.
Why Was OAuth Born?
Back in the day, sharing passwords between apps was a messy, insecure nightmare. Imagine needing to give every app your Facebook password just so it can do something simple—total hassle, right? OAuth came to the rescue, providing a secure way to grant limited access without the password drama. It’s all about making things easier and safer in our connected digital world.
OAuth Actors: The Cast of Characters
Alright, let’s get to know the main players in the OAuth universe. Think of them like characters in your favorite show, each with their own roles to keep the story (or in this case, the data flow) running smoothly.
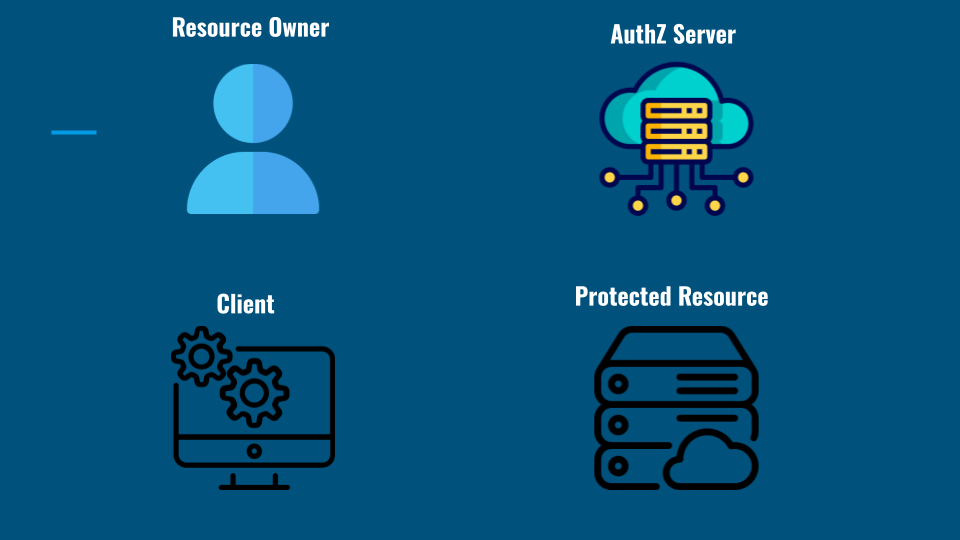
OAuth Actors
1. Resource Owner (You, the User)
- Who They Are: You’re the star of the show. The Resource Owner is the person who owns the data or resources that are being accessed.
- What They Do: You give permission for your data to be accessed by other apps without handing over your password. It’s all about control—deciding who gets to see what.
2. Client (The App)
- Who They Are: This is the app or service that wants to access your data. It could be anything from a social media app to a third-party tool.
- What They Do: The Client requests access to your data from the Resource Owner. It’s like that friend asking to borrow your stuff but in a secure, controlled way.
3. Authorization Server (The Bouncer)
- Who They Are: The gatekeeper of your data. This server is responsible for authenticating the Resource Owner and issuing tokens.
- What They Do: When the Client asks for access, the Authorization Server checks if everything’s legit and then hands out tokens that grant specific access levels.
4. Resource Server
- Who They Are: The actual vault where your data resides. This server holds the protected resources and only allows access via valid tokens.
- What They Do:
- Receive Requests with Tokens: The client (e.g., a web or mobile application) makes requests to the Resource Server, typically including an access token in the
Authorization
header. - Validate Tokens: The Resource Server confirms that the token is valid. This can happen in several ways:
- Local Validation (e.g., verifying a JWT’s signature and claims using a public key).
- Introspection (calling back to the Authorization Server to confirm the token’s status, scope, and expiration).
- Check Scope/Claims: Even if the token is valid, the Resource Server needs to check whether the scope or claims in the token permit the requested action.
- Grant or Deny Access: If the token is valid and the request is authorized, the Resource Server returns the requested data or performs the requested operation. Otherwise, it denies the request.
Resource servers are often the battleground for red team vs blue team simulations, especially when validating token misuse or scope abuse.
Authorization Grant Types: Choosing Your Path
OAuth is versatile, offering different ways (or grant types) for Clients to get those all-important tokens. Each grant type has its own flow and is suited for different scenarios. Let’s break them down:
1. Authorization Code Grant: The VIP Pass
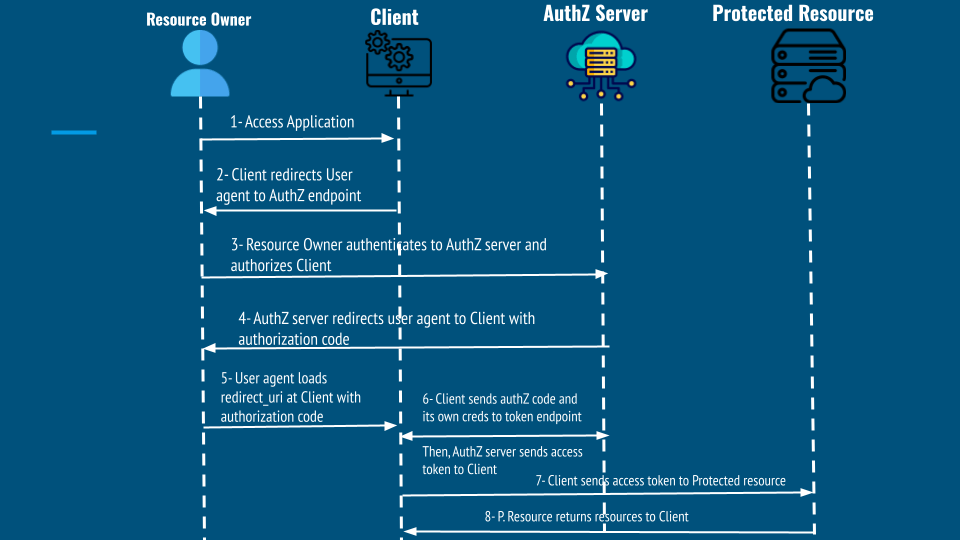
Authorization Code Grant
- When to Use It: Best for server-side applications where the Client can keep a secret (like web apps with a backend server).
- How It Works:
- User Authorization: The user logs in and grants permission to the Client.
- Authorization Code: The Authorization Server sends back a one-time code to the Client.
- Token Exchange: The Client exchanges this code for Access and Refresh Tokens.
2. Implicit Grant: The Quick Pass
- When to Use It: Ideal for single-page applications (SPAs) running in the browser where storing secrets securely is tricky.
- How It Works:
- User Authorization: Similar to the Authorization Code Grant, the user grants permission.
- Access Token Directly: Instead of an authorization code, the Access Token is sent directly to the Client.
3. Resource Owner Password Credentials (ROPC) Grant: The Old School Way
- When to Use It: Only when the Client is highly trusted, like official apps developed by the service itself.
- How It Works:
- User Credentials: The user provides their username and password directly to the Client.
- Token Request: The Client sends these credentials to the Authorization Server to get Access and Refresh Tokens.
- Why It’s Risky: Sharing your password with the Client can be a security nightmare. Use it sparingly and only when absolutely necessary.
4. Client Credentials Grant: The Solo Player
- When to Use It: Perfect for machine-to-machine (M2M) interactions where there’s no user involved, like backend services.
- How It Works:
- Client Authentication: The Client authenticates itself directly with the Authorization Server using its own credentials.
- Token Issuance: It gets an Access Token to access the Resource Server.
- Why It’s Useful: Simplifies scenarios where the Client needs to access its own resources or perform operations without user intervention.
5. Device Code Grant: The Gadget-Friendly Way
- When to Use It: Great for devices with limited input capabilities, like smart TVs or IoT gadgets.
- How It Works:
- Device Requests Code: The device asks the Authorization Server for a device code and user code.
- User Action: The user goes to a separate device (like their phone) to enter the user code and authorize.
- Token Polling: The device periodically checks with the Authorization Server until the user authorizes it and receives the Access Token.
- Why It’s Smooth: It allows users to authorize devices without needing a keyboard or easy input method on the device itself.
6. Refresh Token Grant: The Recharger
- When to Use It: When you need to maintain long-term access without annoying the user with repeated logins.
- How It Works:
- Token Expiry: When the Access Token expires, the Client uses the Refresh Token to request a new Access Token from the Authorization Server.
- Why It’s Essential: Keeps the user experience seamless by refreshing tokens in the background, ensuring continuous access without interruptions.
Tokens: The VIP Passes
- Access Token: This is the golden ticket that lets the app access your data. It’s short-lived to keep things secure.
- Refresh Token: Think of this as a backstage pass. It lets the app get a new Access Token when the old one expires without you having to log in again.
--( OAuth Vulnerabilities )--
While OAuth is a powerhouse for secure authorization, it’s not immune to vulnerabilities. Some vulnerabilities surface only through internal vs external penetration testing, especially when dealing with token leakage across microservices. Understanding these potential weak spots is crucial for building robust applications. Let’s break down the common vulnerabilities associated with each component of OAuth.
1. Vulnerabilities in the OAuth Client
--Client Secret Leakage--
- What’s the Deal?
If you spill your “client secret” out there in public, it’s game over. Attackers can nab it and pretend to be your client app like they’re wearing the world’s best Halloween costume. - How Do People Screw This Up?
- Hard-coding secrets in JavaScript: If you stash the secret in the front-end, guess what—someone’s gonna pop open DevTools and rip it right out.
- Accidental commits: You push the secret to GitHub or another repo “by accident.” Next thing you know, you’re on every hacker’s watch list.
--Insecure Redirect URIs & Open Redirects--
- What’s the Deal?
The redirect_uri
tells the Authorization Server where to bounce the user back with the authorization code or token. If you let attackers mess with the redirect, it’s like letting them wire funds to their own bank account instead of yours. - How Do People Screw This Up?
- They allow wildcard subdomains when choosing the registered
redirect_uri
when the new OAuth client is created at the authorization server. - They have an open redirect on the client’s own site—meaning a “legit” domain can redirect anywhere in the blink of an eye.
- Real-World Impact
- Token Theft: The attacker can capture the authorization code or access token by injecting their own domain or redirect.
- Account Takeover: With a stolen token, attackers can access user data or perform actions on behalf of the user.
--Weak or Missing State Parameter--
- What’s the Deal?
OAuth uses a state
parameter to keep track of who started the login process in the first place. If you skip it or just put something guessable like abc123
, attackers can hijack your sessions or swap codes. - How Do People Screw This Up?
- They don’t use
state
at all (too lazy or forgot). - They use the same
state
value for every session (hey, guess what, that’s basically worthless).
--Lack of PKCE in Public Clients--
- What’s the Deal?
PKCE (Proof Key for Code Exchange) is like a secret handshake for public clients (like mobile apps, SPAs) to prove they’re the rightful owners of an authorization code. If you skip PKCE, you’re basically handing out codes that anyone can redeem if they snag ‘em in transit. - How It Works:
- The client generates a random code verifier before starting the OAuth flow.
- It derives a code challenge (for example, using SHA-256) from that verifier.
- It sends the challenge to the Authorization Server when requesting an authorization code.
- When redeeming the code for tokens, it must send the original verifier. The server checks that
SHA-256(verifier) == code_challenge
. - If they match, the token is issued. If not, it fails.
- How Do People Screw This Up?
- They implement the good ol’ Authorization Code flow but never do the PKCE steps.
- Attackers snoop the code on an unsecured connection or through logs and redeem it themselves.
How to Fix Client-Side Screw-Ups
- Don’t Store Secrets in Plain Sight If it’s a public client, treat it like it has no secrets at all. If you do have a secret, keep it in a secure backend or a vault.
- Lock Down Redirect URIs No wildcards, no sloppy subdomain matching. Stick to https and validate everything.
- Use a Solid
state
to Tackle CSRF Generate a random, super long string and verify it when you get the callback. - PKCE Is Your Best Friend If your client is a mobile or SPA type, use PKCE to block code interception attacks.
- Rotate Secrets Regularly Don’t wait for a crisis to replace your compromised secrets—swap them out on a schedule or when you suspect trouble.
2. Vulnerabilities in the Authorization Server
--Shoddy Input Validation--
- What’s the Deal?
Your Authorization Server needs to be super picky about what clients send its way. If it just trusts every parameter blindly, you’re basically asking for trouble. - How Do People Screw This Up?
- Not verifying that
client_id
and redirect_uri
actually match what’s in the official database. - Letting a shady client trick the server into sending tokens to the wrong place or with the wrong scope.
--Token Expiration Fails--
- What’s the Deal?
Access tokens have a “Use By” date. If your tokens never expire (or have a crazy long lifetime), any stolen token is good until the heat death of the universe. - How Do People Screw This Up?
- They set tokens to never expire “for convenience,” which is a total rookie move.
- They forget or skip refresh token rotation, so if an attacker grabs that refresh token, they can keep re-upping forever.
Locking Down Your Auth Server
- Parameter Validation FTW The server must confirm
redirect_uri
is an exact match for the registered client. No half measures, no partial matches. - Keep Token Lifespans Short Five minutes or so for access tokens is common. Refresh tokens should get rotated every single time they’re used. The old one? Chuck it out.
- Keep an Eye on Logs Set up monitoring so if you see a bunch of weird requests or repeated failures, you know something’s up pronto.
You can take it a step further with this Auth0 misconfiguration checklist, covering tenant isolation, token handling, and dashboard MFA.
3. Vulnerabilities in the Protected Resource (Resource Server)
--Slack Access Control & Scope Checks--
- What’s the Deal?
Even if the Auth Server is on point, the Resource Server has to confirm that the token covers the exact actions being requested. If you don’t check scope, you might be letting a read-only token do write operations. That’s no bueno. - How Do People Screw This Up?
- They never bother to verify the
scope
or role
in the token. - They skip verifying the token’s issuer or audience—so any old token from who-knows-where might pass.
--Token Revocation? What’s That?--
- What’s the Deal?
When somebody logs out or an admin kills a token, the Resource Server should know not to accept it anymore. If it doesn’t, that token can party on like it never got kicked out. - How Do People Screw This Up?
- They don’t bother calling the Auth Server to see if the token’s been revoked.
- They have no real-time revocation list, especially when using stateless JWTs.
Keep Your Resource Server on Lock
- Token Validation Is Non-Negotiable Check the signature, issuer, audience, scope—basically all the claims. If something’s off, toss it out.
- Implement Revocation Checking If you’re using opaque tokens, you can do introspection. If it’s JWT, you might keep a blacklist of revoked tokens or rely on short token lifespans.
- Get Granular with Permissions If your token says “scope: read,” don’t let that user do a delete or update. Keep it tight.
4. Vulnerabilities with Tokens
--Token Leakage in Logs & Browser Storage--
Unvalidated redirects or open metadata endpoints can be exploited for SSRF attacks in cloud environments, especially when tokens leak through misconfigured OAuth flows.
- What’s the Deal?
Tokens are like VIP passes. If they show up in logs, or you stash them in unsafe spots like Local Storage, you’re basically handing out free backstage passes to anyone who finds them. - Why They Leak
- Developers sometimes log entire URLs containing tokens in query parameters or fragments.
- Tokens stored in Local Storage or Session Storage (with no encryption or additional security measures) may be retrieved by malicious JavaScript if the site has XSS (Cross-Site Scripting) vulnerabilities.
- Historically, the Implicit Flow places tokens in the URL hash, which can end up in browser history, logs, or referrer headers.
- How Do People Screw This Up?
- Logging Full URLs
- If your app puts tokens in query parameters or the fragment (
#
), these might appear in web server logs, analytics data, or error monitoring tools. - Any system or person with access to those logs can potentially grab the tokens.
- Storing in Local Storage
- Although it’s easy, Local Storage is accessible via JavaScript. If your site has an XSS vulnerability, attackers can retrieve tokens from Local Storage and use them.
- There’s no built-in expiration or secure flag for Local Storage—it sits there until explicitly cleared.
- Implicit Flow URL Hash
- In the old-school Implicit Flow (mostly discouraged now), tokens come back in the URL fragment (
#access_token=...
). - This can leak through browser history or if the user copies/shares the URL.
--Replay Attacks--
- What’s the Deal?
If some creep intercepts your token on the wire or finds it lying around, they might just send it in again (and again, and again) to do nasty stuff in your name. - How Do People Screw This Up?
- They don’t enforce TLS (HTTPS), or use outdated encryption. Attackers can sniff the network like it’s a free buffet.
- They skip PKCE or other measures to bind tokens to a particular client.
--JWT Shenanigans (Algorithm Confusion)--
- What’s the Deal?
- JWT (JSON Web Token)
- A compact way of conveying identity and authorization info between parties. It consists of a header, payload, and signature.
- The header (
alg
) specifies which signing algorithm (e.g., HS256
, RS256
) was used.
- Algorithm Confusion
- If the server doesn’t strictly enforce which algorithm it expects, a malicious token might say in its header, “I’m signed with HS256,” when in reality, the server’s code tries to verify with an RS256 public key.
- Alternatively, the token might be declared as
alg=none
or something equally dubious, and a poorly implemented server might accept it as valid.
- How Do People Screw This Up?
- Letting the Token Decide
- A naive implementation might trust the
alg
header outright: “Oh, the token says it’s using HS256
? Alright, I’ll just check the signature with HS256
.” - Hackers can trick the server if it expected RS256 but accepted the HS256 signature or even “none.”
- Ignoring RS256 vs. HS256
- RS256 is asymmetric (requires a private key to sign, public key to verify).
- HS256 is symmetric (the same shared secret is used to sign and verify).
- If the server mistakes HS256 for RS256, the attacker might sign the token with any random key (since the server isn’t expecting the correct type of verification).
Token Security Like a Pro
- Guard Those Tokens with Your Life Don’t stash them in local storage unless you’ve really thought it through. Use secure, HttpOnly cookies if possible. Keep them out of logs, error messages, and everything else.
- Short Token Lifetimes Make those tokens expire fast, and refresh them only when needed. That way, if they get stolen, they’re not valid for long.
- Keep JWT Validation Tight Lock in the algorithm you expect. Double-check the signature with the correct public key. No iffy business allowed.
- Use PKCE & State It’s the easiest way to avoid code-stealing drama. PKCE basically ties the authorization code to your specific client instance, so no one else can use it.
Maintaining secure OAuth implementations requires more than one-time audits; continuous penetration testing can help you catch issues like broken redirect handling and stale token use before attackers do.
Next?
Alright, cyber warriors, that’s a wrap on Part One. But don’t go bailing on me yet—this show’s just getting started. Next time, we’re diving headfirst into the nitty-gritty of how OAuth pulls off authentication magic and how you can put social logins under your microscope. If you’ve been itching to probe those “Sign in with Google/Facebook/GitHub” buttons, this is your front-row pass to the wild side.
Stay Connected
If you found this blog helpful, don’t forget to share it with your network and follow for more insights on modern security practices. Until next time, peace out!